Async/Await
The async/await syntax is a special syntax created to help you work with promise objects. It makes your code cleaner and clearer.
When handling Promise, we need to chain the call to the function or variable that returns a Promise using then/catch methods.
Async Keyword
We use the async keyword with a function to represent that the function is an asynchronous function. The async function returns a promise.
async function name(parameter1, parameter2, …paramaterN) {
// statements
}

In this snippet the async keyword is used before the function to represent that the function is asynchronous.
Since this function returns a promise, we can use the chaining method then()
.
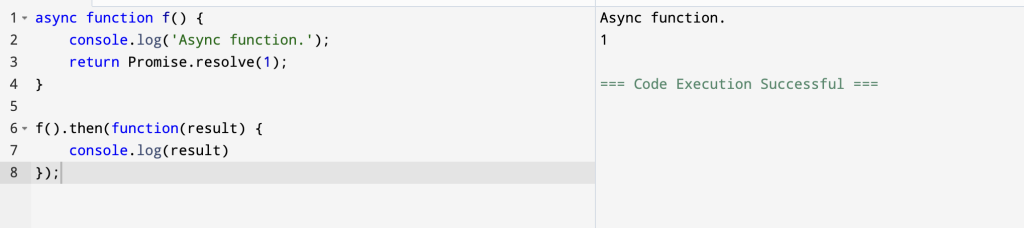
In this snippet f()
function is resolved and the then()
method gets executed.
Await Keyword
The await keyword is used inside the async function to wait for the asynchronous operation.
let result = await promise
The use of await pauses the async function until the promise returns a result (resolve or reject) value.
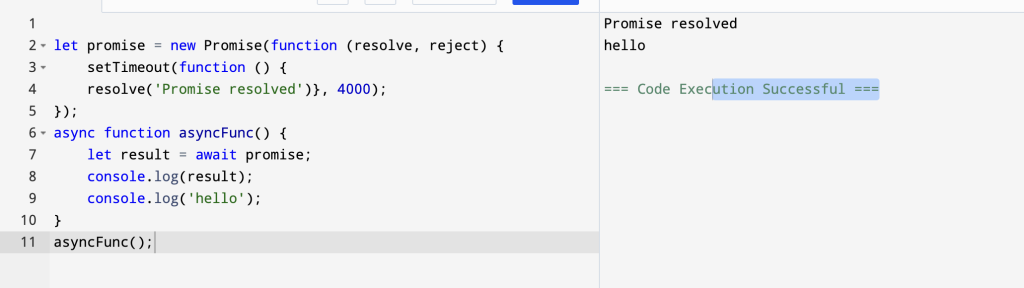
In this snipper a Promise object is created and it gets resolved after 4000 milliseconds. Here, the asyncFunc()
function is written using the async function. The await keyword waits for the promise to be complete (resolve or reject).
Hence, hello is displayed only after promise value is available to the result variable. If await
is not used, hello is displayed before Promise resolved.
Error Handling
While using the async
function, we write the code in a synchronous manner. And you can also use the catch()
method to catch the error.
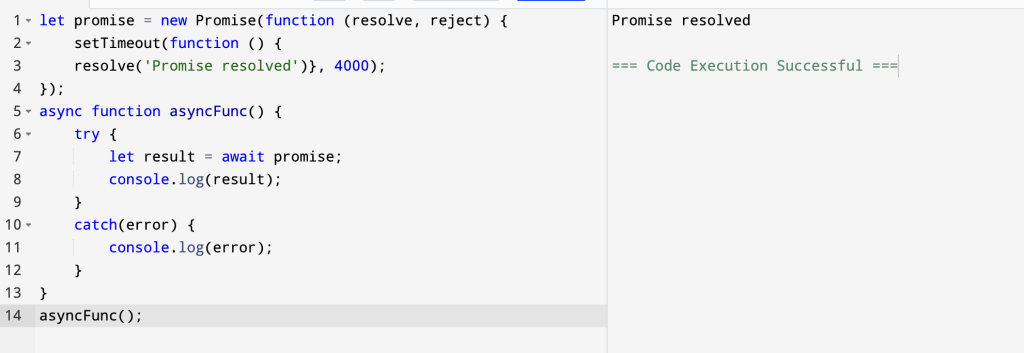
In this snippet try/catch block to handle the errors. If the program runs successfully, it will go to the try block. And if the program throws an error, it will go to the catch block.
Promise vs Async/Await Code Comparison
In the async/await version, the result of the promise is directly assigned to a variable. In the standard promise version, the result of the promise is passed as an argument to the .then() method.
Most of them prefer the async/await version as it looks more straightforward.
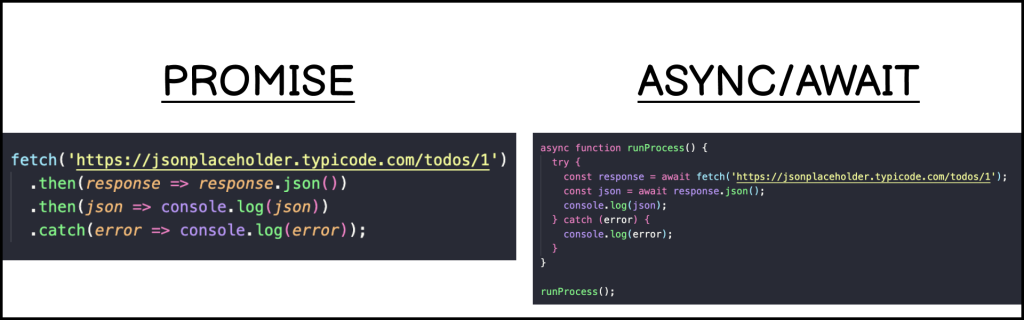
How to Use Async/Await in IIFE and Arrow Functions
An Immediately Invoked Function Expression (IIFE) is a technique used to execute a function immediately as soon as you define it.Unlike regular functions and variables, IIFEs will be removed from the running process once they are executed.
Aside from the standard function, we can also make an asynchronous IIFE. This is useful when we need to run the asynchronous function only once.
(async function () {
try {
const response = await fetch(‘https://jsonplaceholder.typicode.com/todos/1’);
const json = await response.json();
console.log(json);
} catch (error) {
console.log(error);
}
})();
Arrow syntax when creating an asynchronous function
const runProcess = async () => {
try {
const response = await fetch(‘https://jsonplaceholder.typicode.com/todos/1’);
const json = await response.json();
console.log(json);
} catch (error) {
console.log(error);
}
};
runProcess();
Benefits of Using async Function
- The code is more readable than using a callback or a promise.
- Error handling is simpler.
- Debugging is easier.