Error Handling in GraphQL with Apollo Server
Apollo Server is a tool that helps to create GraphQL APIs easily. It makes setting up a GraphQL server simple and fast, allowing to define how data is organized and accessed. Apollo server also has built-n support for handling errors and monitoring performance.
Error handling in GraphQL with Apollo Server helps users clearly understand any issues. When errors are handled correctly, users get clear messages about what went wrong and what they should do next. This makes the API more reliable and provides a better experience for everyone.
default status codes:
Apollo Server is responds with two status codes, which is basically:
200 : This Status code returned even if errors occur during execution
500 : This status code indicates internal server error.
Install apollo server express:
yarn add apollo-server-express
Example:
import { ApolloError, UserInputError } from “apollo-server-express”;
import { Types } from “mongoose”;
getBorrowerbyID: async (_: any, { id }: { id: string }) => {
if (!Types.ObjectId.isValid(id)) {
throw new UserInputError(BORROWER.INVALID_ID);
}
try {
const borrower = await BorrowerModel.findById(id);
if (!borrower) {
throw new ApolloError(BORROWER.BORROWERNOTFOUND);
}
return borrower;
} catch (error) {
throw new ApolloError(BORROWER.INTERNAL_SERVER_ERROR);
}
},
Basic Error handling:
Using ApolloError to return general errors and Handle unexpected issues.
User Input Error:
It validate the user input and if its not validate returns specific error.
if (!Types.ObjectId.isValid(id)) {
throw new UserInputError(BORROWER.INVALID_ID);
}
Format Error:
By default Apollo server Provides a basic error format. To customize the below error format globally, we can use formatError function.
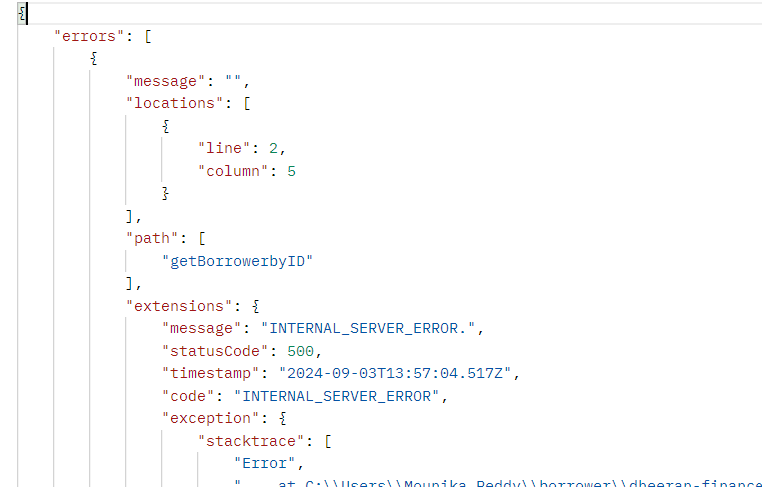
To Add this formatError function inside index.ts file :
import { GraphQLError, GraphQLFormattedError } from ‘graphql’;
const formatError = (error: GraphQLError): GraphQLFormattedError => {
const { message } = error;
return { message };
};
const server = new ApolloServer({
typeDefs,
resolvers,
context,
formatError,
});
After implementing formatError function, error response will include error details inside message field.
Conclusion:
Using ApolloError and UserInputError provides clear error messages, making it easier to understand issues.