Formik: Simplifying Form Management in React
Formik is a popular open-source library designed to simplify form handling in React and React Native applications. Managing forms in React can be tedious because we need to handle form state, validation, and submission manually, which often leads to repetitive and complex code. Formik is used to make this process easier, more organized, and less error-prone, allowing developers to focus on building great user interface.
What is Formik ?
Formik is a lightweight library that helps to manage forms in React by taking care of three key challenges:
- Form State Management : Keeps track of forms inputs, like what the user types, without needs to write a lot of code.
- Validation and Error Handling: Makes it easy to validate user input and display error messages (eg: “Email is invalid” or “password is incorrect”).
- Form Submission: Simplifies the process of submitting form data, including handling loading states and errors during submission.
Why Formik ?
Forms are a critical part of most web applications, from login pages to complex surveys.
- We want to set up state for every input field.
- Write event handle for changes, blurs and submissions.
- Manage validation logic and error message manually.
When we use larger forms with many fields it will be complex to write validation rules but Formik automates these tasks, making your code cleaner, easier to maintain, and faster to write. It’s especially helpful for developers working on the project with multiple forms or those who want to save time without sacrificing quality.
Yup
Yup is used to simplifies validation of the form data using schema-based approach.
Advantage of Formik
Less Code and More Clarity: It reduce the length of code we need to write by handling state, and submission logic. This makes code easier to read and maintain.
Built-in Validation: Formik supports both custom validation and integration with libraries like Yup, a schema validation tool that simplifies complex validation rules.
Field-Level Control: We can able to validate individual fields.
Performance Optimization: Formik minimizes unnecessary re-renders, ensuring forms to perform well even with many fields.
React Ecosystem Integration: Works seamlessly with React, React Native, and UI libraries like Material-UI.
How to Implement ?
Step-1
Install the dependencies like Formik and yup.
npm install formik yup
Step-2
Formik Component <Formik> wraps the form and manages its state. It takes:
- initialValues
- validationSchema
- onSubmit
Step-3
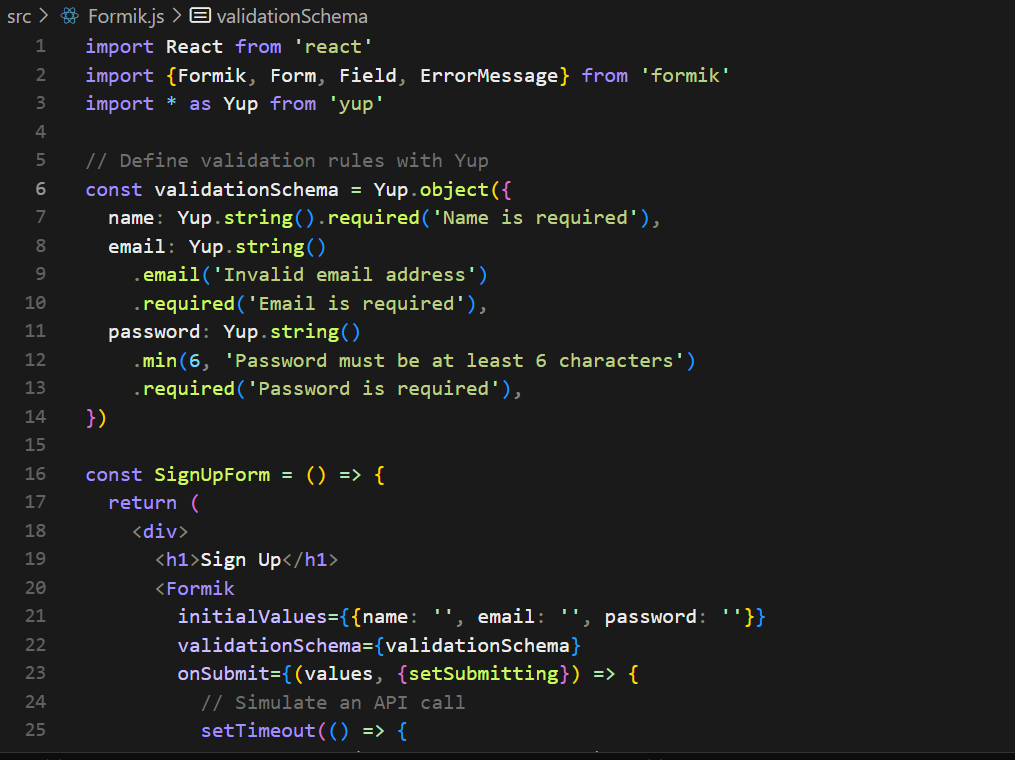
In this snippet Validation Schema is used to validate the email and password and onSubmit it simulates API call.
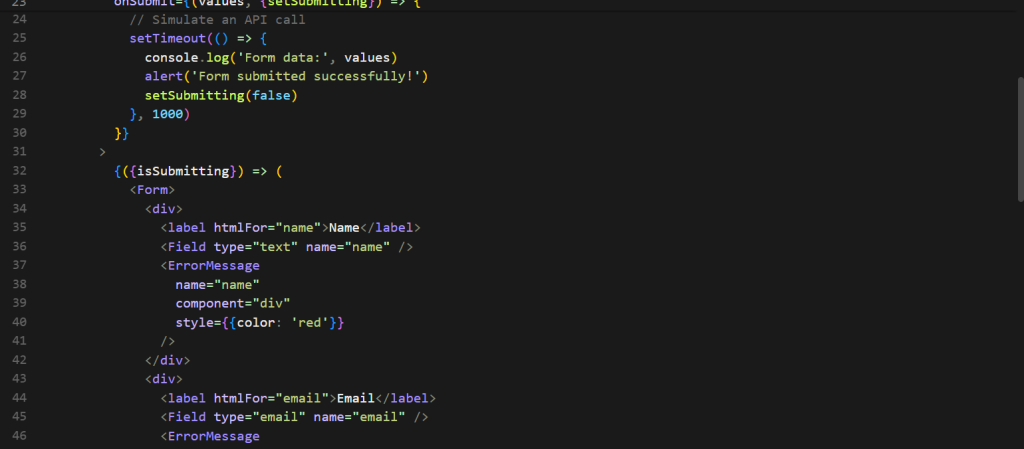
In this snippet form design <Form> component wraps the fields it used to collect the input from the user.
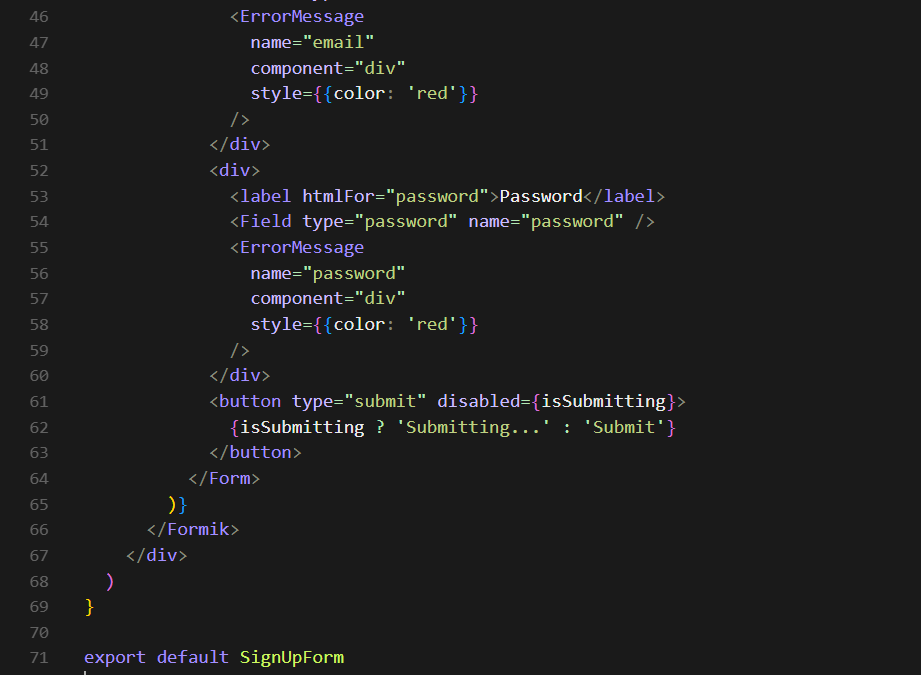
Output:
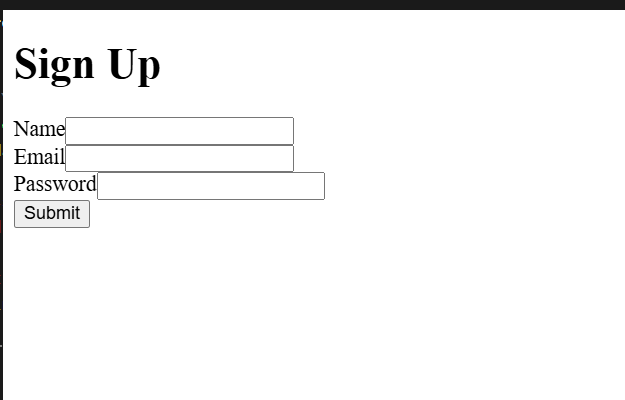
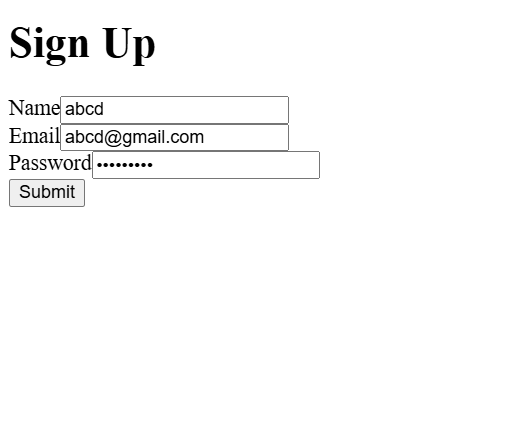
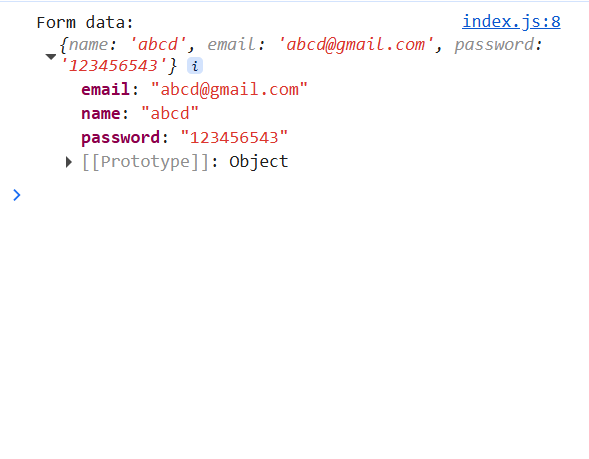
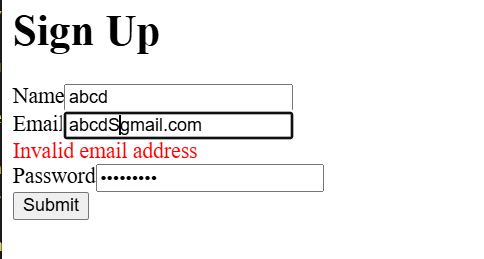
If we enter the invalid email Id it shows the error “invalid email address” by using validation schema.
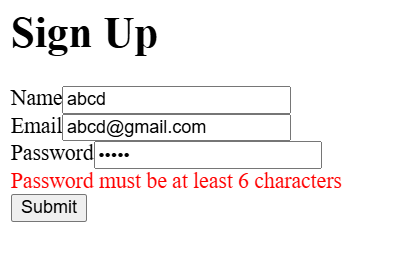
In this snippet shows that password length validation error message.
Formik and Yup are a powerful duo for building forms in React effortlessly. Formik simplifies form state management, validation, and submission, reducing the hassle of writing repetitive code. Paired with Yup’s straightforward schema-based validation, we can create user-friendly form with clear error message and robust input checking in on time.