Email Functionality in Node.Js Application:
In Node.Js applications, we can perform email functionality with the use of “nodemailer” library.
Nodemailer:
It is a comprehensive email module for Node.js, which makes it easy to send emails from your Node.js application. It supports HTML content, attachments, different transport methods (like SMTP, sendmail, etc.), and more.
Transporter:
Transporter is an object created by nodemailer to send emails. It represents the interface to an email server, such as an SMTP server.
Settings details:
When we create a transporter, we need to specify the details of the email server we want to use for sending emails. This includes settings like the service provider (Gmail, Outlook, etc.), authentication details (username and password), and other configuration options. We can also specify host and port details additionally for setting up email service to the transporter.
Note: Some email services will be more secure to allow access from third party applications including nodemailer. In such cases, we need to create separate passkey for nodemailer app and enable the less secure option for email account and hence nodemailer can access the account with the new pass key.
Steps to use nodemailer for email functionality:
1. Install nodemailer with the command:
npm install nodemailer
2. Import nodemailer into the application
const nodemailer = require(‘nodemailer’);
3. Choose email service and account credentials for sending emails
4. Create a transport object using SMTP transport and define the email service and credentials. It is a good practice to store these confidential data in env file and import here.
let transporter = noedmailer.createTransport({
service: ‘Example,
auth: {
user : ‘user@example.com’,
pass: ‘examplepwd’
}
});
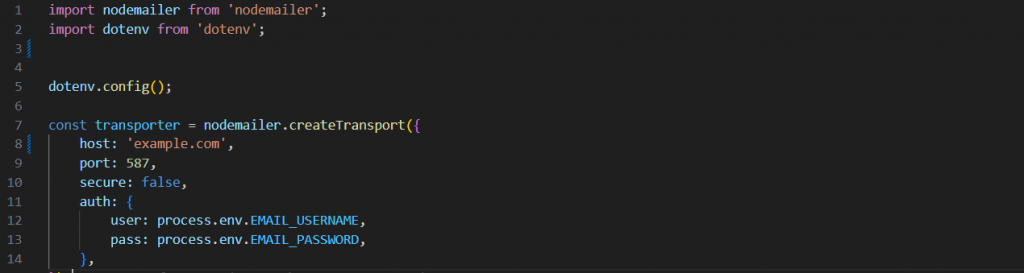
5. Next is to set up email data that need to be transferred. It can be defined as email options.
let mailOption ={
from : ‘Sender Name <sender@example.com>’,
to : ‘recipient email@example.com’,
subject: ‘Hello’
text: ‘mail content’,
html: ‘<b>Hello world</b>’
};
We can also include html files as attachments and images along with the above mail content.
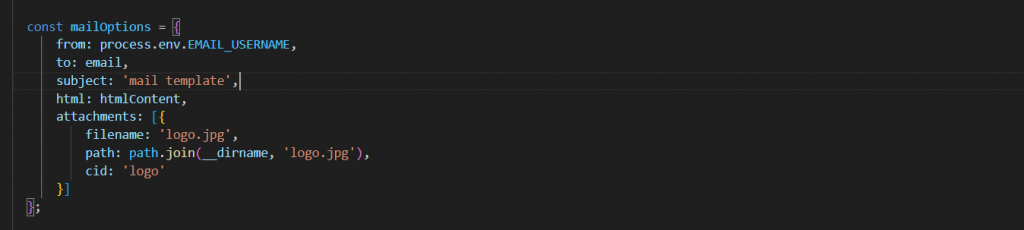
With the above steps and illustrations, we can perform email sending functionality in a more simple way with Node.js applications.