How to secure APIs using JWT Authentication
Nowadays a large number of data is being transmitted over the internet and hence it needs to be secured in a way that no third party can access the sensitive information. To achieve this, JWT(JSON Web Token) is mainly used for securing the transmitted information between parties as a JSON Object.
How JWT Authentication works:
When a user logins, the request is sent to the server with the credentials and server verifies the credentials stored in the database.
If the credentials are valid, the server generates a JWT that contains encoded information (usually user ID, username, and possibly other claims), a signature, and an expiration time. The token is signed using a secret key or a public/private key pair.
The server sends back the JWT to the user as a response to the successful login. This token is stored by the user and includes it in the Authorization header (usually as Bearer <token>) for subsequent requests to the server.
The server validates the token’s signature and checks if it has expired. If the token is valid, the server processes the request and provides access to protected resources. If the token is invalid or expired, the server rejects the request, usually with a 401 Unauthorized response.
Steps to implement JWT in Node project:
1. Install JWT
yarn add jsonwebtoken
2. Setup an authentication middleware:
A middleware is used to verify whether the requests contain valid JWT in the headers and allow requests else it returns a 401 response.
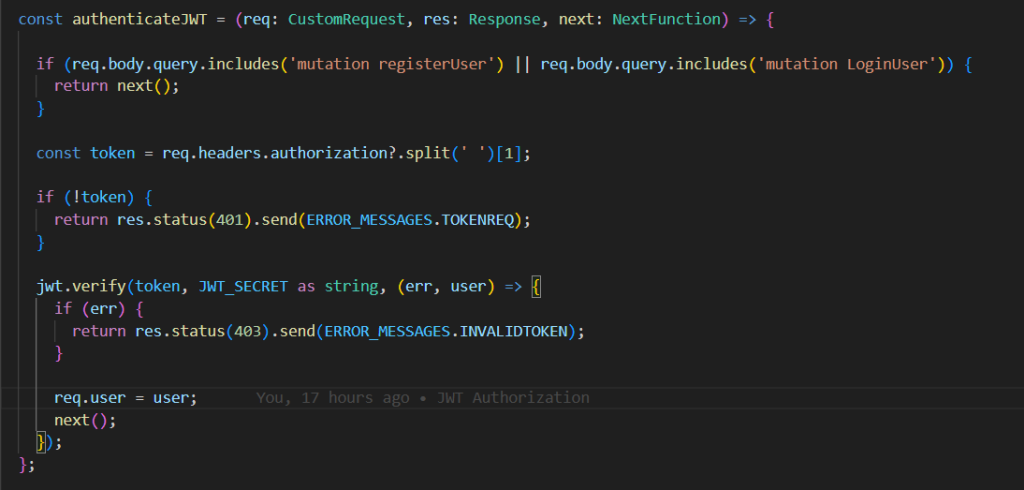
3. Exclude register & login APIs from JWT verification since token will be generated only after login API is requested.
4. Include JWT token generation in login function and return the token in login response.
const token = jwt.sign(
{ userId: user._id, username: user.username },
process.env.JWT_SECRET as string,
{ expiresIn: “8h” }
);
Here the token is valid only for 8hrs from the time it is generated that means the time when the user logs in.
5. Store JWT SECRET key in a common .env file.
The requests from frontend that require authentication should be passed to the server with the JWT in the Authorization header.
In this way, JWT authentication can be implemented for securing the APIs.