Slots in Javascript
Introduction:
In JavaScript, we can create reusable web components, which is a key feature that allows us to avoid repeating code. A crucial aspect of web components is the use of slots, which help manage the content displayed within templates.
What are slots?
Slots are placeholders within a shadow DOM, where you can insert the desired HTML content. They enhance the reusability and flexibility of web components, allowing for dynamic content insertion.
To define a slot we use <slot></slot>. The content is placed between the tags, allowing us to inject external content and dynamically render it within the component.
Example: <slot>Default Content</slot>
Named Slots:
To define multiple slots, we can assign unique names to each one for differentiation.
Example:
<slot name=”header”>Default Header Content</slot>
<slot name=”footer”>Default Footer Content</slot>
I am defining the template with slots.
<template id=”user-card-template”>
<style>
.card {
border: 1px solid #ccc;
max-width: 400px;
}
.header {
color: red;
}
.footer {
color: green;
}
</style>
<div class=”card”>
<div class=”header”><slot name=”header”>Default Header Content</slot></div>
<div class=”footer”><slot name=”footer”>Default Footer Content</slot></div>
</div>
</template>
<script>
class UserCard extends HTMLElement {
constructor() {
super();
const template = document.getElementById(“user-card-template”);
const shadowRoot = this.attachShadow({ mode: “open” });
shadowRoot.appendChild(template.content.cloneNode(true));
}
}
customElements.define(“user-card”, UserCard);
</script>
<user-card>
<h2 slot=”header”>Name: John</h2>
<div slot=”footer”>Footer</div>
</user-card>
<user-card>
<h2 slot=”header”>Name: Jim</h2>
<div slot=”footer”>Footer2 content</div>
</user-card>
Output:
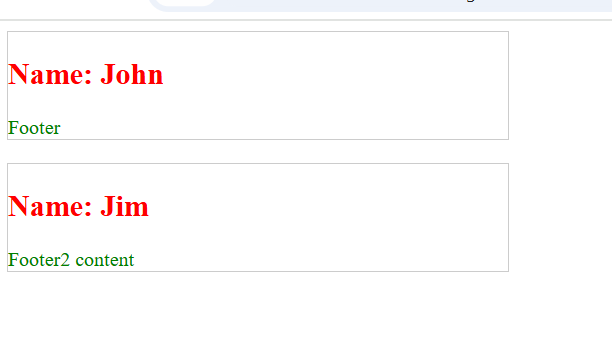
Here two cards are loaded with dynamic contents.
Conclusion:
Slots are very important feature in web components which offer flexibility and control over the content distribution. By using default, named slots we can create a powerful and reusable web components.